When encountering the “ReferenceError: __dirname is not defined in ES module scope” in a Node.js project, it indicates that the __dirname variable is not accessible in the ES module. This commonly occurs when migrating from CommonJS to ES modules and using __dirname in the entry file.
Facing the “ReferenceError: __dirname is not defined in ES module scope” issue can be frustrating, especially during a migration from CommonJS to ES modules in Node. js. This error arises when attempting to access the __dirname variable within the ES module.
However, by understanding the specific nature of this problem and implementing the appropriate solutions, it is possible to effectively resolve this issue and ensure smooth functionality within the ES module scope.
Table of Contents
ToggleIntroduction To Es Modules And __dirname
Encountering the “ReferenceError: __dirname is Not Defined in ES Module Scope” while migrating from CommonJS to ES modules? The error occurs because __dirname and __filename globals are not defined in the ES module scope. To resolve this, you can use the import.
meta. url property to access the current module’s URL.
Commonjs Vs Es Modules
When it comes to working with Node.js, developers have two options for module systems: CommonJS and ES Modules. CommonJS has been the traditional module system for Node.js, but ES Modules are gaining popularity due to their native support in modern browsers. One of the key differences between the two is that CommonJS uses a synchronous approach to loading modules, while ES Modules use an asynchronous approach.The Role Of __dirname
In Node.js, __dirname is a global variable that provides the directory path of the current module. This can be useful for accessing files relative to the current module or for determining the absolute path of the current module. However, when using ES Modules, __dirname is not defined in the module scope by default, which can result in the ReferenceError: __dirname is not defined error. To fix this error, developers can use a workaround by defining a new variable that stores the directory path of the current module. This can be achieved by using the import.meta.url property and the URL API to extract the directory path from the URL. Here’s an example code snippet:import { fileURLToPath } from 'url';
import { dirname } from 'path';
const __filename = fileURLToPath(import.meta.url);
const __dirname = dirname(__filename);
By using this workaround, developers can access the directory path of the current module in ES Modules just like they would in CommonJS.
The Root Cause Of __dirname Not Defined Error
When working with ES modules in Node.js, you may encounter the ReferenceError: __dirname is Not Defined in ES Module Scope. This error occurs when using the __dirname variable in an ES module, as it is not available in the module scope. Understanding the root cause of this error can help in resolving it effectively.
Differences In Global Variables
In CommonJS modules, the __dirname variable represents the directory name of the current module. It is a global variable that is available throughout the module scope. However, ES modules have a different approach to global variables, which leads to the absence of __dirname in the module scope.
Scope Changes In Es Modules
ES modules have a separate scope for variables, which differs from the CommonJS module system. In ES modules, each module has its own scope, and the availability of global variables like __dirname is limited to the CommonJS environment. When attempting to access __dirname in an ES module, the error occurs due to the scope changes introduced by ES modules.
Transitioning From Commonjs To Es Modules
When migrating from CommonJS to ES Modules, developers may encounter the “ReferenceError: __dirname is not defined in ES module scope” issue. This error arises due to the differences in module systems and the unavailability of __dirname in the ES module scope.
Challenges Faced During Migration
During the transition, developers face challenges related to the use of __dirname, which is a Node.js-specific variable not available in ES module scope. This can lead to errors, especially in projects where __dirname is extensively used to resolve file paths.
Refactoring For Es Modules Compatibility
To resolve the “ReferenceError: __dirname is not defined in ES module scope” issue, developers need to refactor the code to make it compatible with ES Modules. This involves identifying instances where __dirname is used and replacing it with alternative solutions that work within the ES module scope, such as using import.meta.url or restructuring the code to eliminate the reliance on __dirname.
Native Alternatives To __dirname In Es Modules
Encountering the error “ReferenceError: __dirname is not defined in ES module scope” can be frustrating when working with ES modules in Node. js. However, there are native alternatives to __dirname such as using import. meta. url and path. resolve() that can help fix this issue.
When migrating an old project from CommonJS to ES modules, you may encounter the error “ReferenceError: __dirname is not defined in ES module scope.” This error occurs because the __dirname global variable, which is available in CommonJS, is not defined in the ES module scope. However, there are native alternatives that you can use in ES modules to achieve similar functionality.
Using Import.meta.url
One native alternative to __dirname in ES modules is to use the import.meta.url property. The import.meta.url property provides the URL of the current module, including the file path. You can extract the directory path from the URL using path manipulation methods. Here’s an example:
const path = require('path');
const currentModuleUrl = new URL(import.meta.url);
const currentModulePath = path.dirname(currentModuleUrl.pathname);
In the above example, we first import the ‘path’ module to access its path manipulation methods. Then, we create a new URL object using the import.meta.url property to get the URL of the current module. Finally, we use the path.dirname() method to extract the directory path from the URL.
Path Manipulation With New Syntax
Another native alternative to __dirname in ES modules is to use the new path manipulation syntax available in ES modules. With this syntax, you can directly manipulate the file path without relying on __dirname. Here’s an example:
import { fileURLToPath } from 'url';
import { dirname } from 'path';
const currentModulePath = dirname(fileURLToPath(import.meta.url));
In the above example, we use the fileURLToPath() function from the ‘url’ module to convert the import.meta.url to a file path. Then, we use the dirname() function from the ‘path’ module to extract the directory path from the file path.
By using these native alternatives, you can overcome the ReferenceError: __dirname is not defined in ES module scope and achieve similar functionality in ES modules.
Third-party Solutions And Polyfills
Encountering the error “ReferenceError: __dirname is not defined in ES module scope” can happen when migrating a project from CommonJS to ES modules. To fix this, you can add the code below: import path from ‘path’; const __dirname = path. resolve(); This will resolve the issue and allow you to use __dirname in your ES module.
If you’re working with ES modules in Node.js, you may have encountered the “ReferenceError: __dirname is not defined in ES module scope” error. This error occurs because the __dirname and __filename globals, which are available in CommonJS, are not defined in the ES module scope. One way to solve this issue is by using third-party solutions and polyfills. These are code snippets or modules that provide a workaround for the missing __dirname and __filename variables.Popular __dirname Polyfills
There are several popular __dirname polyfills available that you can use to fix the “ReferenceError: __dirname is not defined in ES module scope” error. Here are some of them:Package | Description |
---|---|
esm |
A module loader for ES modules that supports __dirname and __filename. |
dynamic-dirs |
A small library that provides dynamic __dirname and __filename variables. |
dirname-call |
A simple module that exports a function that returns the current file’s directory. |
Integrating Polyfills Into Your Project
To use a polyfill, you first need to install it as a dependency in your project using a package manager such as npm or yarn. Once installed, you can import the polyfill module in your code and use it to get the current directory path. Here’s an example of how to use theesm
module to fix the “__dirname is not defined in ES module scope” error:
- Install the
esm
module as a dependency in your project: - Add the following line to the top of your ES module file:
- Use the
__dirname
variable in your code:
npm install esm
import esm from 'esm'; esm(module);
console.log(__dirname);
Credit: github.com
Code Examples: Fixing The __dirname Issue
Encountering the “ReferenceError: __dirname is Not Defined in ES Module Scope” issue while migrating from CommonJS to ES modules? Learn how to fix it by adding the necessary code to resolve this error and ensure smooth module functionality.
Snippet For Import.meta.url
If you are migrating an old project from CommonJS to ES modules and encounter the error “ReferenceError: __dirname is not defined in ES module scope,” you can use the `import.meta.url` property to get the directory name instead of `__dirname`. Here’s an example: “`js import path from ‘path’; const __dirname = path.dirname(new URL(import.meta.url).pathname); “` This code snippet uses the `path` module to extract the directory name from the URL object returned by `import.meta.url`.Implementing Polyfills
Alternatively, you can implement a polyfill to define `__dirname` and `__filename` in ES module scope. Here’s an example: “`js import { fileURLToPath } from ‘url’; import path, { dirname } from ‘path’; const __filename = fileURLToPath(import.meta.url); const __dirname = dirname(__filename); “` This code snippet uses the `url` module to convert the URL object returned by `import.meta.url` to a file path, and then uses the `path` module to extract the directory name from that file path. By implementing this polyfill, you can continue using `__dirname` and `__filename` in your ES module code without encountering the “ReferenceError” issue. Remember to add the following to your `package.json` file to enable ES module support: “`json { “type”: “module” } “` By following these code examples, you can easily fix the “__dirname is not defined in ES module scope” error and continue developing your Node.js applications using ES modules.Common Pitfalls And How To Avoid Them
Encountering the “ReferenceError: __dirname is not defined in ES module scope” can be a common pitfall when migrating projects from CommonJS to ES modules. To avoid this, ensure that you are using the correct syntax and consider using alternative methods to access the directory name within your code.
Debugging Tips
When encountering the “ReferenceError: __Dirname is Not Defined in Es Module Scope” error, it’s important to have some debugging tips in your arsenal to quickly identify and resolve the issue. Here are a few steps you can take to debug this error:- Check your file extension: Ensure that the file extension of the file where the error is occurring is “.js” and not “.mjs”. The “__dirname” variable is only available in CommonJS modules and not in ES modules.
- Review your import statements: Make sure that you are using the correct syntax for importing modules in ES modules. The “__dirname” variable is specific to CommonJS modules and is not available in ES modules by default.
- Verify your build configuration: If you are using a build tool like Babel or Webpack, check that your configuration is set up correctly to handle ES modules. Some build tools may require additional plugins or presets to properly handle the “__dirname” variable.
- Inspect your file structure: Double-check the file structure of your project to ensure that you are referencing the correct file path. Incorrect file paths can lead to the “__dirname” variable not being recognized.
- Utilize console.log: Add console.log statements to your code to output the values of variables and track the execution flow. This can help you identify any issues with the “__dirname” variable and pinpoint where the error is occurring.
Best Practices In Es Modules
To avoid encountering the “ReferenceError: __Dirname is Not Defined in Es Module Scope” error, it’s important to follow best practices when working with ES modules. Here are some guidelines to keep in mind:- Use relative paths: Instead of relying on the “__dirname” variable, use relative paths when referencing files within your project. This ensures that your code is more portable and can be easily moved or deployed without relying on the specific directory structure.
- Consider alternative methods: If you need to access the directory name in ES modules, you can use the “import.meta.url” property to retrieve the URL of the current module and then manipulate it to extract the directory name.
- Stay up to date with ECMAScript specifications: ES modules are part of the ECMAScript standard, and it’s important to stay informed about any updates or changes to the specification. This can help you avoid compatibility issues and ensure that your code adheres to the latest standards.
- Consult official documentation and community resources: When encountering issues with ES modules, consult official documentation and community forums for guidance. These resources can provide valuable insights and solutions to common problems.

Credit: iamwebwiz.medium.com
Future-proofing Your Code
When developing code, it’s important to future-proof it to ensure it remains compatible with evolving standards and technologies. One common issue that developers encounter is the ReferenceError: __Dirname is Not Defined in Es Module Scope error, particularly when migrating from CommonJS to ES modules. Addressing this error is crucial for maintaining the functionality and reliability of your codebase.
Keeping Up With Ecmascript Updates
Staying informed about ECMAScript updates is essential for future-proofing your code. As new features and syntax are introduced, it’s important to adapt your code to leverage these advancements. By keeping abreast of the latest ECMAScript specifications, you can ensure that your code remains compatible with modern development practices and tools.
When To Refactor For New Features
Knowing when to refactor your codebase for new features is critical for future-proofing your applications. As new ECMAScript features are introduced, it’s essential to evaluate whether your existing code can benefit from these enhancements. Refactoring your code to incorporate new features can improve maintainability, performance, and compatibility with the evolving JavaScript ecosystem.
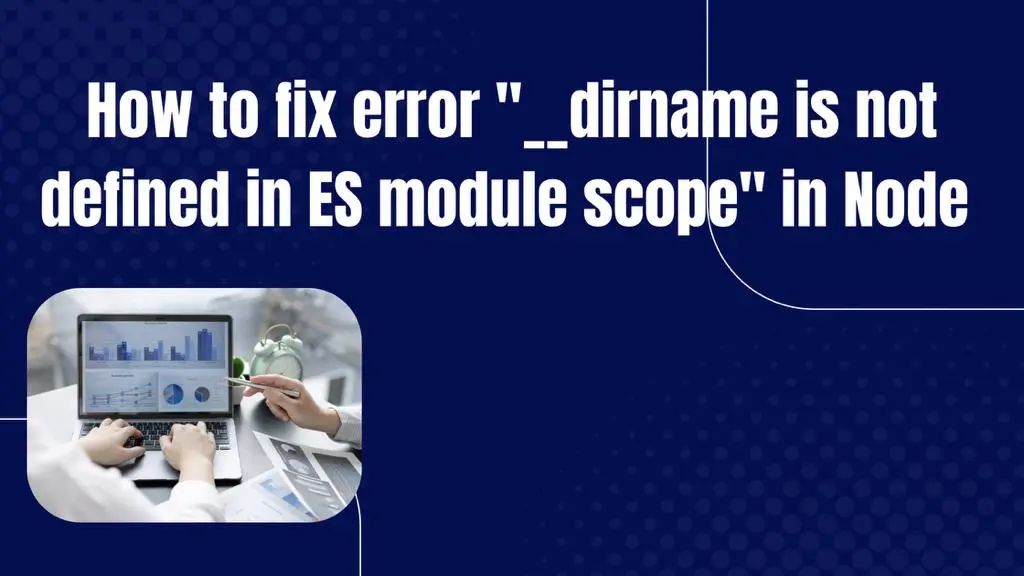
Credit: codebeautify.org
Frequently Asked Questions
What Is The Meaning Of The Error Message “__dirname Is Not Defined In Es Module Scope”?
The error message “__dirname is not defined in ES module scope” occurs when using ES modules in Node. js. It means that the __dirname global variable, which is available in CommonJS, is not defined in the ES module scope. This variable is used to obtain the name of the directory from a given file path.
How Can I Fix The “__dirname Is Not Defined In Es Module Scope” Error?
To fix the “__dirname is not defined in ES module scope” error, you can add the following code:
“`javascript
import path from ‘path’;
const __dirname = path.dirname(new URL(import.meta.url).pathname);
“`
This code sets the __dirname variable to the directory name using the path module in ES modules.
Why Is The __dirname Variable Not Defined In Es Module Scope?
The __dirname variable is not defined in ES module scope because it is a Node. js-specific variable that is available in CommonJS modules. ES modules have their own module system and do not have access to the __dirname variable by default.
This is to encourage the use of more modern and standardized ways of dealing with file paths in ES modules.
Conclusion
To fix the “ReferenceError: __Dirname is Not Defined in Es Module Scope” error, you need to be aware that the __dirname and __filename globals, available in CommonJS, are not defined in the ES module scope. To resolve this, you can use the import.
meta. url property along with the path module to get the directory name. By making this adjustment in your code, you’ll be able to successfully migrate your project from CommonJS to ES modules without encountering this error.